From swapping the characters to reversing the list, there are multiple approaches that can be applied for arriving at a conclusion for a given set of problems.
When we consider the concept of time complexity, it is important for a programmer to reach the output statement as efficiently as possible.
This is why the concept of next permutation was derived.
This concept determines the lexicographically applicable integer in a set of arrays or strings. We have interacted on this concept further in this blog.
Also, if you are interested in learning about the 0 1 Knapsack problem which is similar to the next permutation concept, then make sure to read till the end of the blog.
What do you mean by next permutation?
Before understanding what is the next permutation, let us clarify the definition of permutation.
Basically, permutation is the set of already arranged characters which will be rearranged while running the program.
The definition for next permutation in a set of integers can be defined as the consecutive lexicographically greater permutation of the integers.
To describe the concept more vividly, we can state the following:
“If the permutations were arranged in a specific order, the next permutation within the array of integers will be the lexicographically arranged integer.”
That was the basic definition of the next permutation. Let us now consider the approaches that you can apply for finding the next permutation for a given set of integers.
Which methods can you apply for deriving the next permutation?
In order to derive the next permutation, let us first consider an example to better understand the concept.
Example:
You have been given a set of array,
1, 2, 3
Output:
1, 3, 2
Now, the next permutation for 1, 2, 3 could be the following:
2, 3, 1
1, 3, 2
3, 2, 1
There can be multiple other combinations for deriving the next permutation for this particular array of integers.
But, ultimately for deriving the output you must consider the line of outcome that matches the required output.
Now let us consider the approaches for deriving the next permutation for this given example.
Using the brute force algorithm
The brute approach or the brute algorithm is catered towards deriving all the possible outcomes for the given set of arrays so that the programmer can finalize the outcome that matches the output.
For instance in the above example we can see that the output 1, 3, 2 has been derived in the third line of computation. This is basically how you can employ the brute force algorithm to calculate the next permutation.
Time complexity: The time constraint of the brute force algorithm is that you will have to derive all the possible solutions which can get lengthy.
Using Linear Time Complex
The optimal approach for deriving the next permutation in a given set of arrays uses the linear time complex approach.
The algorithm for this approach traverses the given set of arrays in a linear format from the back of the string. While traversing, the programmer has to figure out the first value of “i”.
In the context of the above example, we can consider that the first linear of “i” while traversing the string backwards will be 2.
This way it will be easier to figure out the index that matches the index that you are trying to derive as the next permutation.
Time complexity: In the linear time complex approach since we are traversing the given array in a backwards manner, hence the time complexity can be iterated as relatively greater than the brute force approach.
When deriving the next permutation on Java, C++ or any other programming languages for that matter, you can consider the native approach which is also known as the brute force approach.
The linear time complex approach is also liberal and optimal for deriving the required results. But, it is both time consuming and the reversal process of the array used in the approach makes it difficult to comprehend for beginner programmers.
Those were the two approaches that you can apply for figuring out the next permutation.
The problem related to next permutation in a given set of arrays can also be approached from a different method or angle.
This approach can provide us insights on the characteristics of the elements such as weight and value. This sort of problem statement is known as knapsack.
Take a look at the 0 1 Knapsack problem for reference.
What is a 0 1 Knapsack problem?
The 0 1 Knapsack problem initiates deriving the weight and the value of elements present within a string. The 0 and 1 in this case are two sets of integers that represent their own respective values.
The integer W in a Knapsack represents the capacity of the maximum value in the problem.
The most important factor to consider in the case of a Knapsack problem is that you cannot divide the elements. You will either have to consider it as a whole or not pick the element at all.
If you encounter the Knapsack problem during your mock tests or other interview formats you can apply the brute force or the recursive approaches.
Both the algorithms follow a clear set of instructions that thoroughly traverse through the string or the array to arrive at a definite output.
The only difference is that in the brute force algorithm you will have to figure out all the probable solutions.
While in the recursive method you will be traversing the string in a reverse manner.
Wrapping Up
The concept of Next Permutation allows the programmers to traverse through the whole string and arrive at the desired output.
This method is more commonly used when there is a time constraint and the programmer has to arrive at the conclusion more efficiently.
Other than that we also have the 0 1 Knapsack problem that can be used to determine the weight and other aspects of the elements present within the problem.
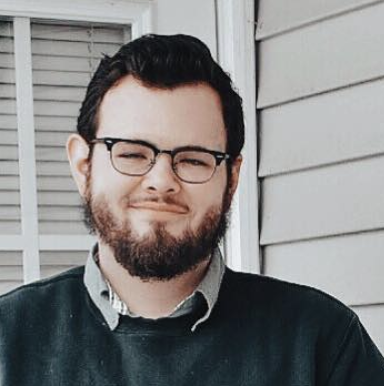
Taylor is a freelance SEO copywriter and blogger. His areas of expertise include technology, pop culture, and marketing.